Exploring the power of Record<K, V> in TypeScript
2 min read
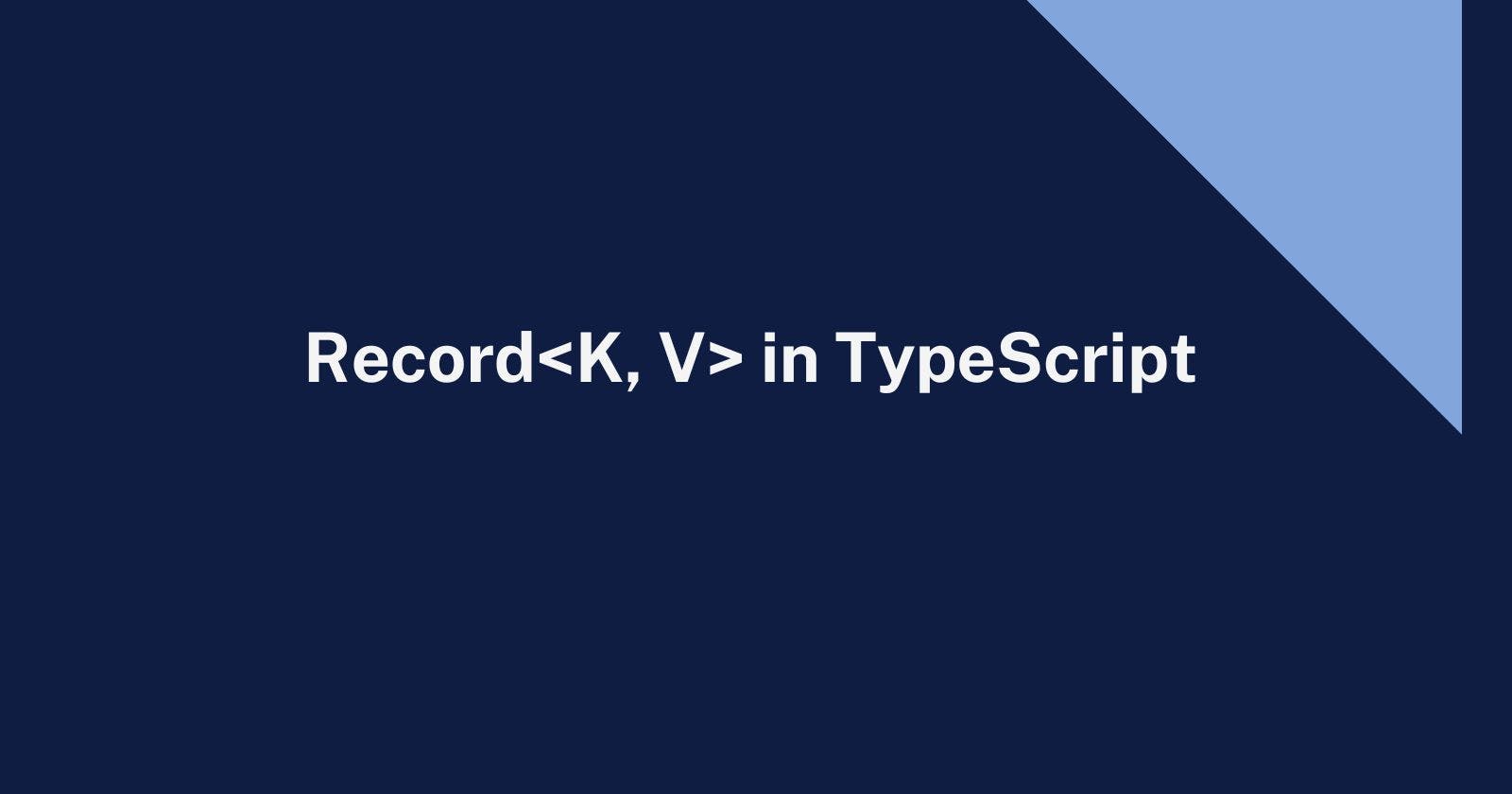
In Javascript almost everything is an object like Arrays, functions, Date, RegExp Promise etc.
You categorise objects
into 2 types:
With Known Properties
: You know properties or methods upfront before even declaring it.Unknown Properties
: You do not know properties or methods before declaring it.
Let's take an example, developer
comes under categorisation where we know all properties before even declaring it.
const developer = {
name: "decpk",
technologies: ["Javscript", "Typescript"],
experience: 8,
getName: function() {
return this.name
}
}
console.log(developer.getName()) // decpk
Let's take another example where data is coming from server
async function callAPI(uri: string) {
try {
const res = await fetch(uri)
const apiResult = await res.json();
return apiResult;
} catch (err) {
// ERROR HANDLING
}
}
callAPI('https://jsonplaceholder.typicode.com/todos/1');
If you hover over apiResult
then you can see that the type is any
.
But why do we care about Known properties
or unknown properties
at all.
If you have known properties then typescript will
infer
types from all those properties but it won't be in case of properties whose type is not known before. In case of unknown properties typescript widen its type toany
So for the above 2 snippet you don't have to specify its type for developer
because typescript is smart enough and it will infer
automatically but you have to specify type for apiResult
and
You don't want to type apiResult
as any
because that would be unsafe code. You still want to make it somewhat type-safe. One way is to type the apiResult
as per the response received from server as:
type Todo = {
userId: number;
id: number;
title: string;
completed: boolean;
};
or other way is to specify more general type which can accomodate any number of properties.
Problem statement
But How would you type an object which can contain any number or type of property.
Object is just a key-value pair. It is also known as Dictionary
so you want to type an object where key is string and value you are not sure then it is better to use Record<K, V>
as
const apiResult: Record<string, unknown> = await res.json();
with this type you are saying that apiResult
will be an object specifically whose key is string
and value is not known or unknown
. So you can't perform any operation until you specify its type.
Hope you learn something new.
Thanks for reading this article.